
- C Programming Tutorial
- C - Home
- C - Overview
- C - Environment Setup
- C - Program Structure
- C - Basic Syntax
- C - Data Types
- C - Variables
- C - Constants
- C - Storage Classes
- C - Operators
- C - Decision Making
- C - Loops
- C - Functions
- C - Scope Rules
- C - Arrays
- C - Pointers
- C - Strings
- C - Structures
- C - Unions
- C - Bit Fields
- C - Typedef
- C - Input & Output
- C - File I/O
- C - Preprocessors
- C - Header Files
- C - Type Casting
- C - Error Handling
- C - Recursion
- C - Variable Arguments
- C - Memory Management
- C - Command Line Arguments
- C Programming useful Resources
- C - Questions & Answers
- C - Quick Guide
- C - Useful Resources
- C - Discussion
- Selected Reading
- UPSC IAS Exams Notes
- Developer's Best Practices
- Questions and Answers
- Effective Resume Writing
- HR Interview Questions
- Computer Glossary
- Who is Who
C Programming - Online Quiz
Following quiz provides Multiple Choice Questions (MCQs) related to C Programming Framework. You will have to read all the given answers and click over the correct answer. If you are not sure about the answer then you can check the answer using Show Answer button. You can use Next Quiz button to check new set of questions in the quiz.
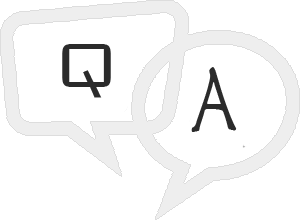
Q 1 - What is the output of the below code snippet?
#include<stdio.h> main() { for()printf("Hello"); }
Answer : D
Explanation
Compiler error, semi colons need to appear though the expressions are optional for the ‘for’ loop.
Q 2 - What is the output of the following program?
#include<stdio.h> void f() { printf(“Hello\n”); } main() { ; }
B - Error, as the function is not called.
C - Error, as the function is defined without its declaration
Answer : A
Explanation
No output, apart from the option (a) rest of the comments against the options are invalid.
Answer : B
Explanation
volatile is the reserved keyword and cannot be used an identifier name.
Q 4 - What is the output of the following program?
#include<stdio.h> main() { char *p = NULL; printf("%c", *p); }
Answer : D
Explanation
It is invalid to access the NULL address hence giving run time error.
Q 5 - What is the output of the following program?
#include<stdio.h> main() { int x; float y; y = x = 7.5; printf("x=%d y=%f", x, y); }
Answer : A
Explanation
‘x’ gets the integral value from 7.5 which is 7 and the same is initialized to ‘y’.
Q 6 - The types of linkages are,
A - Internal linkage and External linkage
B - Internal linkage, External linkage and None linkage
Answer : B
Explanation
- External Linkage-> A global, non-static variables and functions.
- Internal Linkage-> A static variables and functions with file scope.
- None Linkage-> A Local variables.
Q 7 - For a structure, if a variable behave as a pointer then from the given below operators which operator can be used to access data of the structure via the variable pointer?
Answer : C
Explanation
For a structure, Dot(.) operator can be used to access the data using normal structure variable and arrow (->)can be used to access the data using pointer variable.
Q 8 - Choose the correct statement that is a combination of these two statements,
Statement 1: char *p; Statement 2: p = (char*) malloc(100);
B - char *p = (char*)malloc(100);
Answer : B
Explanation
ptr = (data type *)malloc(size);
The given above code is a prototype of malloc() function, where ptr indicates the pointer.
char *p = (char*)malloc(100);
In this code, “*p” is a pointer of type char and malloc() function allocates the memory for char.
Q 9 - Choose the correct order of evaluation,
A - Relational Arithmetic Logical Assignment
B - Arithmetic Relational Logical Assignment
Answer : B
Explanation
As per the operators preference.
Q 10 - What will be the output of the given below code?
#include<stdio.h> int main() { const int *ptr = &i; char str[] = "Welcome"; s = str; while(*s) printf("%c", *s++); return 0; }
Answer : A
Explanation
Although, char str[] = "Welcome"; and s = str;, the program will print the value of s.
#include<stdio.h> int main() { const int *ptr = &i; char str[] = "Welcome"; s = str; while(*s) printf("%c", *s++); return 0; }