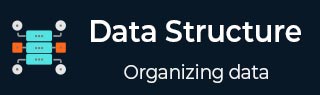
- Data Structures & Algorithms
- DSA - Home
- DSA - Overview
- DSA - Environment Setup
- Algorithm
- DSA - Algorithms Basics
- DSA - Asymptotic Analysis
- DSA - Greedy Algorithms
- DSA - Divide and Conquer
- DSA - Dynamic Programming
- Data Structures
- DSA - Data Structure Basics
- DSA - Data Structures and Types
- DSA - Array Data Structure
- Linked Lists
- DSA - Linked List Basics
- DSA - Doubly Linked List
- DSA - Circular Linked List
- Stack & Queue
- DSA - Stack
- DSA - Expression Parsing
- DSA - Queue
- Searching Techniques
- DSA - Linear Search
- DSA - Binary Search
- DSA - Interpolation Search
- DSA - Hash Table
- Sorting Techniques
- DSA - Sorting Algorithms
- DSA - Bubble Sort
- DSA - Insertion Sort
- DSA - Selection Sort
- DSA - Merge Sort
- DSA - Shell Sort
- DSA - Quick Sort
- Graph Data Structure
- DSA - Graph Data Structure
- DSA - Depth First Traversal
- DSA - Breadth First Traversal
- Tree Data Structure
- DSA - Tree Data Structure
- DSA - Tree Traversal
- DSA - Binary Search Tree
- DSA - AVL Tree
- DSA - Red Black Trees
- DSA - B Trees
- DSA - B+ Trees
- DSA - Splay Trees
- DSA - Spanning Tree
- DSA - Tries
- DSA - Heap
- Recursion
- DSA - Recursion Basics
- DSA - Tower of Hanoi
- DSA - Fibonacci Series
- DSA Useful Resources
- DSA - Questions and Answers
- DSA - Quick Guide
- DSA - Useful Resources
- DSA - Discussion
- Selected Reading
- UPSC IAS Exams Notes
- Developer's Best Practices
- Questions and Answers
- Effective Resume Writing
- HR Interview Questions
- Computer Glossary
- Who is Who
Data Structures Algorithms Online Quiz
Following quiz provides Multiple Choice Questions (MCQs) related to Data Structures Algorithms. You will have to read all the given answers and click over the correct answer. If you are not sure about the answer then you can check the answer using Show Answer button. You can use Next Quiz button to check new set of questions in the quiz.
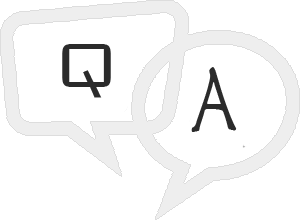
Q 1 - In a min-heap:
A - parent nodes have values greater than or equal to their childs
B - parent nodes have values less than or equal to their childs
Answer : A
Explanation
In a min heap, parents always have lesser or equal values than that of their childs.
Q 2 - What data structure is used for breadth first traversal of a graph?
Answer : A
Explanation
Queue is used for breadth first traversal whereas stack is used for depth first traversal.
Q 3 - Minimum number of moves required to solve a Tower of Hanoi puzzle is
Answer : C
Explanation
Minimum number of moves required to solve a Tower of Hanoi puzzle is 2n - 1. Where n is the number of disks. If the number of disks is 3, then minimum number of moves required are 23 - 1 = 7
Answer : C
Explanation
Every connected graph at least has one spanning tree.
Q 5 - Which of the below given series is Non-Increasing Order −
Answer : C
Explanation
A sequence of values is said to be in non-increasing order, if the successive element is less than or equal to its previous element in the sequence.
Q 6 - In binary heap, whenever the root is removed then the rightmost element of last level is replaced by the root. Why?
A - It is the easiest possible way.
B - To make sure that it is still complete binary tree.
Answer : B
Explanation
A binary heap (whether max or min) has to satisfy the property of complete binary tree at all times.
Q 7 - In doubly linked lists
A - a pointer is maintained to store both next and previous nodes.
B - two pointers are maintained to store next and previous nodes.
Answer : B
Explanation
One pointer variable can not store more than one address values.
Q 8 - In the deletion operation of max heap, the root is replaced by
A - next available value in the left sub-tree.
B - next available value in the right sub-tree.
Answer : D
Explanation
Regardless of being min heap or max heap, root is always replaced by last element of the last level.
Q 9 - From a complete graph, by removing maximum _______________ edges, we can construct a spanning tree.
Answer : A
Explanation
We can remove maximum e-n+1
edges to get a spanning tree from complete graph. Any more deletion of edges will lead the graph to be disconnected.
Q 10 - If the data collection is in sorted form and equally distributed then the run time complexity of interpolation search is −
Answer : D
Explanation
Runtime complexity of interpolation search algorithm is Ο(log (log n)) as compared to Ο(log n) of BST in favourable situations.