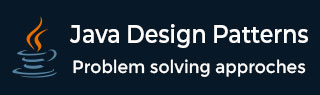
- Design Patterns Tutorial
- Design Patterns - Home
- Design Patterns - Overview
- Design Patterns - Factory Pattern
- Abstract Factory Pattern
- Design Patterns - Singleton Pattern
- Design Patterns - Builder Pattern
- Design Patterns - Prototype Pattern
- Design Patterns - Adapter Pattern
- Design Patterns - Bridge Pattern
- Design Patterns - Filter Pattern
- Design Patterns - Composite Pattern
- Design Patterns - Decorator Pattern
- Design Patterns - Facade Pattern
- Design Patterns - Flyweight Pattern
- Design Patterns - Proxy Pattern
- Chain of Responsibility Pattern
- Design Patterns - Command Pattern
- Design Patterns - Interpreter Pattern
- Design Patterns - Iterator Pattern
- Design Patterns - Mediator Pattern
- Design Patterns - Memento Pattern
- Design Patterns - Observer Pattern
- Design Patterns - State Pattern
- Design Patterns - Null Object Pattern
- Design Patterns - Strategy Pattern
- Design Patterns - Template Pattern
- Design Patterns - Visitor Pattern
- Design Patterns - MVC Pattern
- Business Delegate Pattern
- Composite Entity Pattern
- Data Access Object Pattern
- Front Controller Pattern
- Intercepting Filter Pattern
- Service Locator Pattern
- Transfer Object Pattern
- Design Patterns Resources
- Design Patterns - Questions/Answers
- Design Patterns - Quick Guide
- Design Patterns - Useful Resources
- Design Patterns - Discussion
- Selected Reading
- UPSC IAS Exams Notes
- Developer's Best Practices
- Questions and Answers
- Effective Resume Writing
- HR Interview Questions
- Computer Glossary
- Who is Who
Design Patterns Mock Test
This section presents you various set of Mock Tests related to Design Patterns Framework. You can download these sample mock tests at your local machine and solve offline at your convenience. Every mock test is supplied with a mock test key to let you verify the final score and grade yourself.
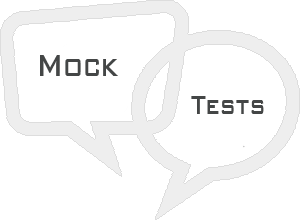
Design Patterns Mock Test I
Q 1 - Which of the following is true about design patterns?
Answer : D
Explanation
Design patterns represent the best practices used by experienced object-oriented software developers. Design patterns are solutions to general problems that software developers faced during software development. These solutions were obtained by trial and error by numerous software developers over quite a substantial period of time.
Q 2 - What is Gang of Four (GOF)?
B - Gang of Four (GOF) is a name of a book on Design Patterns.
Answer : A
Explanation
In 1994, four authors Erich Gamma, Richard Helm, Ralph Johnson and John Vlissides published a book titled Design Patterns - Elements of Reusable Object-Oriented Software which initiated the concept of Design Pattern in Software development. These authors are collectively known as Gang of Four (GOF).
Q 3 - Which of the following is correct list of classifications of design patterns.
A - Creational, Structural and Behavioral patterns.
B - Executional, Structural and Behavioral patterns.
Answer : A
Explanation
Design patterns can be classified in three categories: Creational, Structural and Behavioral patterns.
Q 4 - Which of the following is correct about Creational design patterns.
A - These design patterns are specifically concerned with communication between objects.
Answer : B
Explanation
Creational design patterns provide a way to create objects while hiding the creation logic, rather than instantiating objects directly using new opreator.
Q 5 - Which of the following is correct about Structural design patterns.
A - These design patterns are specifically concerned with communication between objects.
Answer : C
Explanation
Structural design patterns concern class and object composition. Concept of inheritance is used to compose interfaces and define ways to compose objects to obtain new functionalities.
Q 6 - Which of the following is correct about Behavioral design patterns.
A - These design patterns are specifically concerned with communication between objects.
Answer : A
Explanation
Behavioral design patterns are specifically concerned with communication between objects.
Q 7 - Which of the following is correct about Factory design pattern.
A - This type of design pattern comes under creational pattern.
B - Factory pattern creates object without exposing the creation logic to the client.
C - Factory pattern refers to newly created object using a common interface.
Answer : D
Explanation
Factory pattern is one of most used design pattern in Java. This type of design pattern comes under creational pattern as this pattern provides one of the best ways to create an object. In Factory pattern, we create object without exposing the creation logic to the client and refer to newly created object using a common interface.
Q 8 - Which of the following is correct about Abstract Factory design pattern.
A - This type of design pattern comes under creational pattern.
B - Abstract Factory patterns work around a super-factory which creates other factories.
Answer : D
Explanation
Abstract Factory patterns work around a super-factory which creates other factories. This factory is also called as factory of factories. This type of design pattern comes under creational pattern as this pattern provides one of the best ways to create an object. In Abstract Factory pattern an interface is responsible for creating a factory of related objects without explicitly specifying their classes. Each generated factory can give the objects as per the Factory pattern.
Q 9 - Which of the following is correct about Singleton design pattern.
A - This type of design pattern comes under creational pattern.
Answer : D
Explanation
Singleton pattern is one of the simplest design patterns in Java. This type of design pattern comes under creational pattern as this pattern provides one of the best ways to create an object.This pattern involves a single class which is responsible to create an object while making sure that only single object gets created. This class provides a way to access its only object which can be accessed directly without need to instantiate the object of the class.
Answer : A
Explanation
true. It is possible to get a clone of singleton object. Throw exception within the body of clone() method to prevent cloning.
Q 11 - If we serialize a singleton object and deserialize it then the result object will be same.
Answer : B
Explanation
false. Deserializing a serialized object will yield a different object.
Answer : A
Explanation
true. Wrapper classes like Integer, Boolean uses Decorator pattern.
Answer : A
Explanation
true. Each java application uses Runtime as a single object.
Answer : B
Explanation
true. Integer.valueOf() returns a Integer instance representing the specified int value.
Answer : B
Explanation
true. Event handling frameworks like swing, awt use Observer Pattern.
Q 16 - Which of the following describes the Builder pattern correctly?
A - This pattern builds a complex object using simple objects and using a step by step approach.
B - This pattern refers to creating duplicate object while keeping performance in mind.
C - This pattern is used when creation of object directly is costly.
Answer : A
Explanation
Builder pattern builds a complex object using simple objects and using a step by step approach. This builder is independent of other objects.
Q 17 - Which of the following describes the Bridge pattern correctly?
A - This pattern builds a complex object using simple objects and using a step by step approach.
B - This pattern refers to creating duplicate object while keeping performance in mind.
C - This pattern is used when creation of object directly is costly.
Answer : D
Explanation
Bridge pattern is used when we need to decouple an abstraction from its implementation so that the two can vary independently.
Q 18 - Which of the following describes the Prototype pattern correctly?
A - This pattern builds a complex object using simple objects and using a step by step approach.
B - This pattern refers to creating duplicate object while keeping performance in mind.
C - This pattern works as a bridge between two incompatible interfaces.
Answer : B
Explanation
Prototype pattern refers to creating duplicate object while keeping performance in mind.
Q 19 - Which of the following describes the Adapter pattern correctly?
A - This pattern builds a complex object using simple objects and using a step by step approach.
B - This pattern refers to creating duplicate object while keeping performance in mind.
C - This pattern works as a bridge between two incompatible interfaces.
Answer : C
Explanation
Adapter pattern works as a bridge between two incompatible interfaces. This pattern involves a single class which is responsible to join functionalities of independent or incompatible interfaces.
Q 20 - Which of the following describes the Filter pattern correctly?
A - This pattern builds a complex object using simple objects and using a step by step approach.
B - This pattern refers to creating duplicate object while keeping performance in mind.
Answer : B
Explanation
Filter pattern or Criteria pattern is a design pattern that enables developers to filter a set of objects using different criteria and chaining them in a decoupled way through logical operations.
Q 21 - Which of the following pattern builds a complex object using simple objects and using a step by step approach?
Answer : A
Explanation
Builder Pattern builds a complex object using simple objects and using a step by step approach. This builder is independent of other objects.
Q 22 - Which of the following pattern refers to creating duplicate object while keeping performance in mind?
Answer : C
Explanation
Prototype pattern refers to creating duplicate object while keeping performance in mind.
Q 23 - Which of the following pattern works as a bridge between two incompatible interfaces?
Answer : B
Explanation
Adapter pattern works as a bridge between two incompatible interfaces. This pattern involves a single class which is responsible to join functionalities of independent or incompatible interfaces.
Q 24 - Which of the following pattern is used when we need to decouple an abstraction from its implementation so that the two can vary independently?
Answer : A
Explanation
Bridge pattern is used when we need to decouple an abstraction from its implementation so that the two can vary independently.
Q 25 - Which of the following pattern is used when creation of object directly is costly?
Answer : A
Explanation
Prototype pattern is used when creation of object directly is costly.
Answer Sheet
Question Number | Answer Key |
---|---|
1 | D |
2 | A |
3 | A |
4 | B |
5 | C |
6 | A |
7 | D |
8 | D |
9 | D |
10 | A |
11 | B |
12 | A |
13 | A |
14 | B |
15 | B |
16 | A |
17 | D |
18 | B |
19 | C |
20 | B |
21 | A |
22 | C |
23 | B |
24 | A |
25 | A |