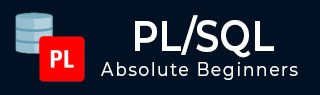
- PL/SQL Tutorial
- PL/SQL - Home
- PL/SQL - Overview
- PL/SQL - Environment
- PL/SQL - Basic Syntax
- PL/SQL - Data Types
- PL/SQL - Variables
- PL/SQL - Constants and Literals
- PL/SQL - Operators
- PL/SQL - Conditions
- PL/SQL - Loops
- PL/SQL - Strings
- PL/SQL - Arrays
- PL/SQL - Procedures
- PL/SQL - Functions
- PL/SQL - Cursors
- PL/SQL - Records
- PL/SQL - Exceptions
- PL/SQL - Triggers
- PL/SQL - Packages
- PL/SQL - Collections
- PL/SQL - Transactions
- PL/SQL - Date & Time
- PL/SQL - DBMS Output
- PL/SQL - Object Oriented
- PL/SQL Useful Resources
- PL/SQL - Questions and Answers
- PL/SQL - Quick Guide
- PL/SQL - Useful Resources
- PL/SQL - Discussion
- Selected Reading
- UPSC IAS Exams Notes
- Developer's Best Practices
- Questions and Answers
- Effective Resume Writing
- HR Interview Questions
- Computer Glossary
- Who is Who
PL/SQL Online Quiz
Following quiz provides Multiple Choice Questions (MCQs) related to PL/SQL. You will have to read all the given answers and click over the correct answer. If you are not sure about the answer then you can check the answer using Show Answer button. You can use Next Quiz button to check new set of questions in the quiz.
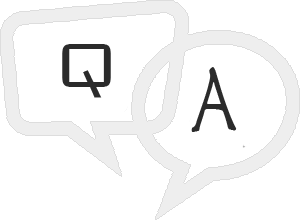
Q 1 - Which of the following is not true about the execution section of a PL/SQL block?
A - It should have more than one executable line of code.
B - It may have just a NULL command to indicate that nothing should be executed.
Answer : A
Q 2 - What will be the output of the following code snippet?
DECLARE a number (2) := 21; b number (2) := 10; BEGIN IF ( a <= b ) THEN dbms_output.put_line(a); END IF; IF ( b >= a ) THEN dbms_output.put_line(a); END IF; IF ( a <> b ) THEN dbms_output.put_line(b); END IF; END;
Answer : C
Q 3 - What is wrong in the following code snippet?
DECLARE x number := 1; BEGIN LOOP dbms_output.put_line(x); x := x + 1; IF x > 10 THEN exit; END IF; dbms_output.put_line('After Exit x is: ' || x); END;
B - The IF statement is not required.
Answer : C
Q 4 - Consider a variable named greetings declared as −
greetings varchar2(11) := 'Hello World';
What will be the output of the code snippet
dbms_output.put_line ( SUBSTR (greetings, 7, 5));
Answer : A
Q 5 - What would be the output of the following code?
DECLARE num number; fn number; FUNCTION fx(x number) RETURN number IS f number; BEGIN IF x=0 THEN f := 1; ELSE f := x * fx(x-1); END IF; RETURN f; END; BEGIN num:= 5; fn := fx(num); dbms_output.put_line(fn); END;
Answer : D
Q 6 - Consider the exception declared as −
emp_exception1 EXCEPTION;
Which of the following statement will correctly call the exception in a PL/SQL block?
A - IF c_id <= 0 THEN ex_invalid_id;
B - IF c_id <= 0 THEN CALL ex_invalid_id;
Answer : C
Q 7 - Observe the syntax given below −
CREATE [OR REPLACE ] TRIGGER trigger_name {BEFORE | AFTER | INSTEAD OF } {INSERT [OR] | UPDATE [OR] | DELETE} [OF col_name] ON table_name [REFERENCING OLD AS o NEW AS n] [FOR EACH ROW] WHEN (condition) DECLARE Declaration-statements BEGIN Executable-statements EXCEPTION Exception-handling-statements END;
Which of the following holds true for the [REFERENCING OLD AS o NEW AS n] clause?
B - OLD and NEW references are not available for table level triggers.
Answer : D
Q 8 - All objects placed in a package specification are called
Answer : A
Q 9 - The collection method LAST
A - Returns the last (largest) index numbers in a collection that uses integer subscripts.
B - Returns the number of elements that a collection currently contains.
Answer : A
Q 10 - Which of the following is not true about the comparison methods?
A - These are used for comparing objects.
C - The Order methods implement some internal logic for comparing two objects.
Answer : B
Explanation
The Map method is a function implemented in such a way that its value depends upon the value of the attributes.