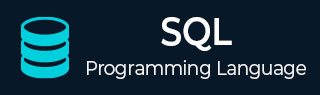
- SQL Tutorial
- SQL - Home
- SQL - Overview
- SQL - RDBMS Concepts
- SQL - Databases
- SQL - Syntax
- SQL - Data Types
- SQL - Operators
- SQL - Expressions
- SQL Database
- SQL - Create Database
- SQL - Drop Database
- SQL - Select Database
- SQL - Rename Database
- SQL - Show Database
- SQL - Backup Database
- SQL Table
- SQL - Create Table
- SQL - Show Tables
- SQL - Rename Table
- SQL - Truncate Table
- SQL - Clone Tables
- SQL - Temporary Tables
- SQL - Alter Tables
- SQL - Drop Table
- SQL - Delete Table
- SQL - Constraints
- SQL Queries
- SQL - Insert Query
- SQL - Select Query
- SQL - Select Into
- SQL - Insert Into Select
- SQL - Update Query
- SQL - Delete Query
- SQL - Sorting Results
- SQL Views
- SQL - Create Views
- SQL - Update Views
- SQL - Drop Views
- SQL - Rename Views
- SQL Operators and Clauses
- SQL - Where Clause
- SQL - Top Clause
- SQL - Distinct Clause
- SQL - Order By Clause
- SQL - Group By Clause
- SQL - Having Clause
- SQL - AND & OR
- SQL - BOOLEAN (BIT) Operator
- SQL - LIKE Operator
- SQL - IN Operator
- SQL - ANY, ALL Operators
- SQL - EXISTS Operator
- SQL - CASE
- SQL - NOT Operator
- SQL - NOT EQUAL
- SQL - IS NULL
- SQL - IS NOT NULL
- SQL - NOT NULL
- SQL - BETWEEN Operator
- SQL - UNION Operator
- SQL - UNION vs UNION ALL
- SQL - INTERSECT Operator
- SQL - EXCEPT Operator
- SQL - Aliases
- SQL Joins
- SQL - Using Joins
- SQL - Inner Join
- SQL - Left Join
- SQL - Right Join
- SQL - Cross Join
- SQL - Full Join
- SQL - Self Join
- SQL - Delete Join
- SQL - Update Join
- SQL - Left Join vs Right Join
- SQL - Union vs Join
- SQL Keys
- SQL - Unique Key
- SQL - Primary Key
- SQL - Foreign Key
- SQL - Composite Key
- SQL - Alternate Key
- SQL Indexes
- SQL - Indexes
- SQL - Create Index
- SQL - Drop Index
- SQL - Show Indexes
- SQL - Unique Index
- SQL - Clustered Index
- SQL - Non-Clustered Index
- Advanced SQL
- SQL - Wildcards
- SQL - Comments
- SQL - Injection
- SQL - Hosting
- SQL - Min & Max
- SQL - Null Functions
- SQL - Check Constraint
- SQL - Default Constraint
- SQL - Stored Procedures
- SQL - NULL Values
- SQL - Transactions
- SQL - Sub Queries
- SQL - Handling Duplicates
- SQL - Using Sequences
- SQL - Auto Increment
- SQL - Date & Time
- SQL - Cursors
- SQL - Common Table Expression
- SQL - Group By vs Order By
- SQL - IN vs EXISTS
- SQL - Database Tuning
- SQL Function Reference
- SQL - Date Functions
- SQL - String Functions
- SQL - Aggregate Functions
- SQL - Numeric Functions
- SQL - Text & Image Functions
- SQL - Statistical Functions
- SQL - Logical Functions
- SQL - Cursor Functions
- SQL - JSON Functions
- SQL - Conversion Functions
- SQL - Datatype Functions
- SQL Useful Resources
- SQL - Questions and Answers
- SQL - Quick Guide
- SQL - Useful Functions
- SQL - Useful Resources
- SQL - Discussion
- Selected Reading
- UPSC IAS Exams Notes
- Developer's Best Practices
- Questions and Answers
- Effective Resume Writing
- HR Interview Questions
- Computer Glossary
- Who is Who
SQL - Comments
In Programming, a comment is a technique to hide a line of code from the compiler. It is also defined as an annotation and program-readable explanation of a computer program, added with the purpose of making the source code easier for humans to understand in a better way.
SQL-Comments
Comments are used to explain the section of the SQL statement; or to stop the execution of the statement. So, whenever a line(s) of code is marked as a comment in a program, it is not executed.
Let us say we are performing a SQL query to display the contents of a table. But if the user does not understand the query, then we can utilize the comments to explain the idea or the query.
There are three types of comments in SQL, let’s see them in detail −
- Single-line comment.
- Multi-line comment.
- In line comment.
Single-line comment
A single line comment is one that ends in a single line and begins with '—'(double hyphen), and the text after the '—'(double hyphen) is not executable.
Syntax
Following is the syntax of the single-line comment.
--fetch all the table details --another comment. --SQL SELECT Query. SELECT * from table_name
Multi-line comment
A multi-line comment is a text that starts in one line and ends in different line, as well as text which is between these (/*…. */) symbols is known as multiline comment.
Syntax
Following is the syntax of the multi-line comment.
/* this is first comment This is second comment Multi line comment*/ SELECT * FROM table_name;
Inline-comment
An inline comment is the same as a multiline comment that is enclosed between /* and /*; but, an inline comment is one that is present at the same line where the SQL query is provided.
Syntax
Following is then syntax of the inline comment.
SELECT * FROM table_name; /*customers*/
Example
In the following example, we define a SQL query that will create a table and display its contents, as well as provide all types of comments to explain the query in a better way.
--CREATING A TABLE CREATE TABLE Customerss(ID INT NOT NULL, NAME VARCHAR(50), AGE INT NOT NULL); /* INSERTING COLUMN'S VALUE IN A TABLE NAMELY CUSTMERS */ INSERT INTO Customerss VALUES(01, 'Tutorialspoint', 10); INSERT INTO Customerss VALUES(02, 'Tutorix', 03); SELECT * FROM Customerss; /* DISPLAYING TABLE DETAILS */
When we run the above SQL query, we get the column values, however the comment line is not executable, as we can see in the table below −
+----+----------------+-----+ | ID | NAME | AGE | +----+----------------+-----+ | 1 | Tutorialspoint | 10 | | 2 | Tutorix | 3 | +----+----------------+-----+