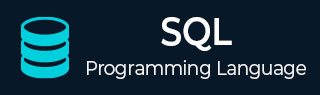
- SQL Tutorial
- SQL - Home
- SQL - Overview
- SQL - RDBMS Concepts
- SQL - Databases
- SQL - Syntax
- SQL - Data Types
- SQL - Operators
- SQL - Expressions
- SQL Database
- SQL - Create Database
- SQL - Drop Database
- SQL - Select Database
- SQL - Rename Database
- SQL - Show Database
- SQL - Backup Database
- SQL Table
- SQL - Create Table
- SQL - Show Tables
- SQL - Rename Table
- SQL - Truncate Table
- SQL - Clone Tables
- SQL - Temporary Tables
- SQL - Alter Tables
- SQL - Drop Table
- SQL - Delete Table
- SQL - Constraints
- SQL Queries
- SQL - Insert Query
- SQL - Select Query
- SQL - Select Into
- SQL - Insert Into Select
- SQL - Update Query
- SQL - Delete Query
- SQL - Sorting Results
- SQL Views
- SQL - Create Views
- SQL - Update Views
- SQL - Drop Views
- SQL - Rename Views
- SQL Operators and Clauses
- SQL - Where Clause
- SQL - Top Clause
- SQL - Distinct Clause
- SQL - Order By Clause
- SQL - Group By Clause
- SQL - Having Clause
- SQL - AND & OR
- SQL - BOOLEAN (BIT) Operator
- SQL - LIKE Operator
- SQL - IN Operator
- SQL - ANY, ALL Operators
- SQL - EXISTS Operator
- SQL - CASE
- SQL - NOT Operator
- SQL - NOT EQUAL
- SQL - IS NULL
- SQL - IS NOT NULL
- SQL - NOT NULL
- SQL - BETWEEN Operator
- SQL - UNION Operator
- SQL - UNION vs UNION ALL
- SQL - INTERSECT Operator
- SQL - EXCEPT Operator
- SQL - Aliases
- SQL Joins
- SQL - Using Joins
- SQL - Inner Join
- SQL - Left Join
- SQL - Right Join
- SQL - Cross Join
- SQL - Full Join
- SQL - Self Join
- SQL - Delete Join
- SQL - Update Join
- SQL - Left Join vs Right Join
- SQL - Union vs Join
- SQL Keys
- SQL - Unique Key
- SQL - Primary Key
- SQL - Foreign Key
- SQL - Composite Key
- SQL - Alternate Key
- SQL Indexes
- SQL - Indexes
- SQL - Create Index
- SQL - Drop Index
- SQL - Show Indexes
- SQL - Unique Index
- SQL - Clustered Index
- SQL - Non-Clustered Index
- Advanced SQL
- SQL - Wildcards
- SQL - Comments
- SQL - Injection
- SQL - Hosting
- SQL - Min & Max
- SQL - Null Functions
- SQL - Check Constraint
- SQL - Default Constraint
- SQL - Stored Procedures
- SQL - NULL Values
- SQL - Transactions
- SQL - Sub Queries
- SQL - Handling Duplicates
- SQL - Using Sequences
- SQL - Auto Increment
- SQL - Date & Time
- SQL - Cursors
- SQL - Common Table Expression
- SQL - Group By vs Order By
- SQL - IN vs EXISTS
- SQL - Database Tuning
- SQL Function Reference
- SQL - Date Functions
- SQL - String Functions
- SQL - Aggregate Functions
- SQL - Numeric Functions
- SQL - Text & Image Functions
- SQL - Statistical Functions
- SQL - Logical Functions
- SQL - Cursor Functions
- SQL - JSON Functions
- SQL - Conversion Functions
- SQL - Datatype Functions
- SQL Useful Resources
- SQL - Questions and Answers
- SQL - Quick Guide
- SQL - Useful Functions
- SQL - Useful Resources
- SQL - Discussion
- Selected Reading
- UPSC IAS Exams Notes
- Developer's Best Practices
- Questions and Answers
- Effective Resume Writing
- HR Interview Questions
- Computer Glossary
- Who is Who
SQL - Having Clause
SQL HAVING clause is similar to the WHERE clause; they are both used to filter rows in a table based on conditions. However, the HAVING clause was included in SQL to filter grouped rows instead of single rows. These rows are grouped together by the GROUP BY clause, so, the HAVING clause must always be followed by the GROUP BY clause. It can be used with aggregate functions, whereas the WHERE clause cannot.
HAVING clause can also contain multiple conditions in it to filter the grouped results. These conditions are separated by various operators like AND, OR etc.
Syntax
Following is the basic syntax of the HAVING clause in SQL −
SELECT column1, column2, aggregate_function(column) FROM table_name GROUP BY column1, column2 HAVING condition;
The following code block shows the position of the HAVING Clause in a query.
SELECT FROM WHERE GROUP BY HAVING ORDER BY
HAVING clause with ORDER BY clause
We can use the HAVING clause with the GROUP BY clause to filter groups of rows that meet certain conditions. It is used to apply a filter to the result set after the aggregation has been performed.
Example
Assume we have created a table with name CUSTOMERS in SQL database using CREATE TABLE statement as shown below −
CREATE TABLE CUSTOMERS ( ID INT NOT NULL, NAME VARCHAR (20) NOT NULL, AGE INT NOT NULL, GENDER VARCHAR (25), SALARY DECIMAL (18, 2), PRIMARY KEY (ID) );
Following query inserts values into this table using the INSERT statement −
insert INTO CUSTOMERS VALUES(1, 'Ramesh', 25, 'Male', 2000.00); insert INTO CUSTOMERS VALUES(2, 'Ramesh', 25, 'Male', 1500.00); insert INTO CUSTOMERS VALUES(3, 'kaushik', 25, 'Female', 2000.00); insert INTO CUSTOMERS VALUES(4, 'kaushik', 20, 'Male', 6500.00); insert INTO CUSTOMERS VALUES(5, 'Hardik', 25, 'Male', 8500.00); insert INTO CUSTOMERS VALUES(6, 'Komal', 20, 'Female', 4500.00); insert INTO CUSTOMERS VALUES(7, 'Muffy', 25, 'Male', 10000.00);
If we verify the contents of the CUSTOMERS table using the SELECT statement, we can observe the inserted records as shown below −
SELECT * from CUSTOMERS; +----+---------+-----+--------+----------+ | ID | NAME | AGE | GENDER | SALARY | +----+---------+-----+--------+----------+ | 1 | Ramesh | 25 | Male | 2000.00 | | 2 | Ramesh | 25 | Male | 1500.00 | | 3 | kaushik | 25 | Female | 2000.00 | | 4 | kaushik | 20 | Male | 6500.00 | | 5 | Hardik | 25 | Male | 8500.00 | | 6 | Komal | 20 | Female | 4500.00 | | 7 | Muffy | 25 | Male | 10000.00 | +----+---------+-----+--------+----------+
Now, we are trying to retrieve all records from the CUSTOMERS table where the sum of their salary is less than 4540, ordered by their name in ascending order −
SELECT NAME, SUM(SALARY) as total_salary FROM CUSTOMERS GROUP BY NAME HAVING SUM(SALARY) < 4540 ORDER BY NAME
Output
The result produced is as follows −
+--------+--------------+ | NAME | total_salary | +--------+--------------+ | Komal | 4500.00 | | Ramesh | 3500.00 | +--------+--------------+
HAVING clause with COUNT() function
The HAVING clause can be used with the COUNT() function to filter groups based on the number of rows they contain.
Example
Following is the query, which would display a record where count of similar age is greater than or equal to 3.
SELECT AGE FROM CUSTOMERS GROUP BY age HAVING COUNT(age) >= 3
Output
This would produce the following result −
+-----+ | AGE | +-----+ | 25 | +-----+
HAVING clause with AVG() function
The HAVING clause can also be used with the AVG() function to filter groups based on the average value of a specified column.
Example
Now, we are trying to retrieve the gender of the customers whose average salary is greater than 5240 −
SELECT gender, AVG(salary) as avg_salary FROM customers GROUP BY gender HAVING AVG(salary) > 5240
Output
Following is the output of the above query −
+--------+-------------+ | gender | avg_salary | +--------+-------------+ | Male | 5700.000000 | +--------+-------------+
HAVING clause with MAX() function
We can also use the HAVING clause with MAX() function to filter groups based on the maximum value of a specified column.
Example
In here, we are trying to retrieve the gender of the customers whose maximum salary is less than 5240 −
SELECT gender, MAX(salary) as max_salary FROM customers GROUP BY gender HAVING MAX(salary) < 5240
Output
The result obtained is as follows −
+--------+-------------+ | gender | max_salary | +--------+-------------+ | Female | 4500.00 | +--------+-------------+