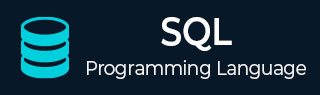
- SQL Tutorial
- SQL - Home
- SQL - Overview
- SQL - RDBMS Concepts
- SQL - Databases
- SQL - Syntax
- SQL - Data Types
- SQL - Operators
- SQL - Expressions
- SQL Database
- SQL - Create Database
- SQL - Drop Database
- SQL - Select Database
- SQL - Rename Database
- SQL - Show Database
- SQL - Backup Database
- SQL Table
- SQL - Create Table
- SQL - Show Tables
- SQL - Rename Table
- SQL - Truncate Table
- SQL - Clone Tables
- SQL - Temporary Tables
- SQL - Alter Tables
- SQL - Drop Table
- SQL - Delete Table
- SQL - Constraints
- SQL Queries
- SQL - Insert Query
- SQL - Select Query
- SQL - Select Into
- SQL - Insert Into Select
- SQL - Update Query
- SQL - Delete Query
- SQL - Sorting Results
- SQL Views
- SQL - Create Views
- SQL - Update Views
- SQL - Drop Views
- SQL - Rename Views
- SQL Operators and Clauses
- SQL - Where Clause
- SQL - Top Clause
- SQL - Distinct Clause
- SQL - Order By Clause
- SQL - Group By Clause
- SQL - Having Clause
- SQL - AND & OR
- SQL - BOOLEAN (BIT) Operator
- SQL - LIKE Operator
- SQL - IN Operator
- SQL - ANY, ALL Operators
- SQL - EXISTS Operator
- SQL - CASE
- SQL - NOT Operator
- SQL - NOT EQUAL
- SQL - IS NULL
- SQL - IS NOT NULL
- SQL - NOT NULL
- SQL - BETWEEN Operator
- SQL - UNION Operator
- SQL - UNION vs UNION ALL
- SQL - INTERSECT Operator
- SQL - EXCEPT Operator
- SQL - Aliases
- SQL Joins
- SQL - Using Joins
- SQL - Inner Join
- SQL - Left Join
- SQL - Right Join
- SQL - Cross Join
- SQL - Full Join
- SQL - Self Join
- SQL - Delete Join
- SQL - Update Join
- SQL - Left Join vs Right Join
- SQL - Union vs Join
- SQL Keys
- SQL - Unique Key
- SQL - Primary Key
- SQL - Foreign Key
- SQL - Composite Key
- SQL - Alternate Key
- SQL Indexes
- SQL - Indexes
- SQL - Create Index
- SQL - Drop Index
- SQL - Show Indexes
- SQL - Unique Index
- SQL - Clustered Index
- SQL - Non-Clustered Index
- Advanced SQL
- SQL - Wildcards
- SQL - Comments
- SQL - Injection
- SQL - Hosting
- SQL - Min & Max
- SQL - Null Functions
- SQL - Check Constraint
- SQL - Default Constraint
- SQL - Stored Procedures
- SQL - NULL Values
- SQL - Transactions
- SQL - Sub Queries
- SQL - Handling Duplicates
- SQL - Using Sequences
- SQL - Auto Increment
- SQL - Date & Time
- SQL - Cursors
- SQL - Common Table Expression
- SQL - Group By vs Order By
- SQL - IN vs EXISTS
- SQL - Database Tuning
- SQL Function Reference
- SQL - Date Functions
- SQL - String Functions
- SQL - Aggregate Functions
- SQL - Numeric Functions
- SQL - Text & Image Functions
- SQL - Statistical Functions
- SQL - Logical Functions
- SQL - Cursor Functions
- SQL - JSON Functions
- SQL - Conversion Functions
- SQL - Datatype Functions
- SQL Useful Resources
- SQL - Questions and Answers
- SQL - Quick Guide
- SQL - Useful Functions
- SQL - Useful Resources
- SQL - Discussion
- Selected Reading
- UPSC IAS Exams Notes
- Developer's Best Practices
- Questions and Answers
- Effective Resume Writing
- HR Interview Questions
- Computer Glossary
- Who is Who
SQL - IS NOT NULL
The IS NOT NULL query in SQL is used to fetch all the rows that contain non-null values in a column.
A NULL value in the context of the relational database model denotes an unidentified value. If we think of a theoretical way, the NULL value still points to an unknown value, but this unknown value is not equal to a value of zero or a field with empty fields.
Null value in SQL
The SQL NULL is the term used to represent a missing value. A NULL value in a table is a value in a field that appears to be blank.
A field with a NULL value is a field with no value. It is very important to understand that a NULL value is different than a zero value or a field that contains spaces. For checking null values we can use two basic operators
- IS NULL
- IS NOT NULL
IS NOT NULL in SQL
Retrieving the rows with non-NULL values in a column is done using the IS NOT NULL operator. When it is used, the condition is met when either the column contains a value that is not null or when the expression that comes before the IS NOT NULL does not evaluate to null.
Syntax
Following is the syntax for IS NOT NULL −
SELECT column_names FROM table_name WHERE column_name IS NOT NULL;
Example
Let’s consider a table named "Car" that we are going to create in our database and which contains some null values in the fields. Execute the below query to create a table
SQL> CREATE TABLE Car ( ID INT NOT NULL, NAME VARCHAR (20) NOT NULL, OWNER CHAR (25), PRICE DECIMAL (18, 2) NOT NULL, PRIMARY KEY (ID) );
Now we are going to populate the above-created table by using the below query.
SQL> INSERT INTO Car (ID,NAME,OWNER,PRICE) VALUES (1, 'Bmw', 'SRK', 2000.00 ); INSERT INTO Car (ID,NAME,OWNER,PRICE) VALUES (2, 'Audi', 'RAM', 3000.00 ); INSERT INTO Car (ID,NAME,OWNER,PRICE) VALUES (3, 'RX100', NULL, 4000.00 ); INSERT INTO Car (ID,NAME,OWNER,PRICE) VALUES (4, 'Benz', NULL, 5000.00 );
Verification
To check whether the table is created or not, let’s execute the below query.
SQL> SELECT * FROM Car;
On executing it, it will display a table as shown below −
+----+-------+-------+---------+ | ID | NAME | OWNER | PRICE | +----+-------+-------+---------+ | 1 | Bmw | SRK | 2000.00 | | 2 | Audi | RAM | 3000.00 | | 3 | RX100 | NULL | 4000.00 | | 4 | Benz | NULL | 5000.00 | +----+-------+-------+---------+
IS NOT NULL with SELECT statement
We can use IS NOT NULL operator with a SELECT statement to filter rows based on whether a particular column contains a non-NULL value.
Example
In the following example, we are going to show how the IS NOT NULL condition is going to be used to select rows with non-NULL values.
SQL> SELECT * FROM Car WHERE OWNER IS NOT NULL;
Output
On executing the above query, it will generate an output as shown below −
+----+------+-------+---------+ | ID | NAME | OWNER | PRICE | +----+------+-------+---------+ | 1 | Bmw | SRK | 2000.00 | | 2 | Audi | RAM | 3000.00 | +----+------+-------+---------+
IS NOT NULL with COUNT() function
We can also use the IS NOT NULL operator with the COUNT() function in SQL to count the number of rows that do not contain NULL values in a particular column. This function is utilized along with the SQL SELECT command.
Syntax
Following is the syntax for COUNT() function −
SELECT COUNT(column_name) FROM table_name WHERE condition;
Example
To determine how many rows are having non-NULL values, use the COUNT() method along with IS NOT NULL. Execute the following query that is shown below −
SQL> SELECT COUNT(*) FROM CAR WHERE OWNER IS NOT NULL;
Output
On executing the above query, it will generate an output as shown below −
+----------+ | COUNT(*) | +----------+ | 2 | +----------+
IS NOT NULL with DELETE statement
We can also use the DELETE statement with IS NOT NULL operator to delete all rows that do not contain NULL values in a particular column.
Example
In the following example, we are going to use IS NOT NULL along with the delete statement to delete the rows that contain non-NULL values. Execute the below query to delete the non-NULL values.
SQL> DELETE FROM Car WHERE OWNER IS NOT NULL;
Verification
To check whether the non-NULL values are removed from the table, execute the following query −
SQL> SELECT * FROM Car;
On executing the above query, it will generate the following output as shown below −
+----+-------+-------+---------+ | ID | NAME | OWNER | PRICE | +----+-------+-------+---------+ | 3 | RX100 | NULL | 4000.00 | | 4 | Benz | NULL | 5000.00 | +----+-------+-------+---------+
IS NOT NULL with UPDATE statement
We can use the UPDATE statement with the "IS NOT NULL" operator in SQL to update the value of a column for all rows that contain a non-NULL value in a particular column.
Example
In this case, we are going to check how we are going to use "is not null" in an update statement. Consider the following table "Car" in our database and run the following query to see how the update statement was used.
SQL> UPDATE Car SET OWNER = 'DHRUVA' WHERE OWNER IS NOT NULL;
Verification
Run the below query to verify whether the table has been updated or not −
SQL> SELECT * FROM Car;
On executing the above query, it will generate the following output as shown below −
+----+-------+--------+---------+ | ID | NAME | OWNER | PRICE | +----+-------+--------+---------+ | 1 | Bmw | DHRUVA | 2000.00 | | 2 | Audi | DHRUVA | 3000.00 | | 3 | RX100 | NULL | 4000.00 | | 4 | Benz | NULL | 5000.00 | +----+-------+--------+---------+