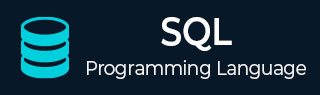
- SQL Tutorial
- SQL - Home
- SQL - Overview
- SQL - RDBMS Concepts
- SQL - Databases
- SQL - Syntax
- SQL - Data Types
- SQL - Operators
- SQL - Expressions
- SQL Database
- SQL - Create Database
- SQL - Drop Database
- SQL - Select Database
- SQL - Rename Database
- SQL - Show Database
- SQL - Backup Database
- SQL Table
- SQL - Create Table
- SQL - Show Tables
- SQL - Rename Table
- SQL - Truncate Table
- SQL - Clone Tables
- SQL - Temporary Tables
- SQL - Alter Tables
- SQL - Drop Table
- SQL - Delete Table
- SQL - Constraints
- SQL Queries
- SQL - Insert Query
- SQL - Select Query
- SQL - Select Into
- SQL - Insert Into Select
- SQL - Update Query
- SQL - Delete Query
- SQL - Sorting Results
- SQL Views
- SQL - Create Views
- SQL - Update Views
- SQL - Drop Views
- SQL - Rename Views
- SQL Operators and Clauses
- SQL - Where Clause
- SQL - Top Clause
- SQL - Distinct Clause
- SQL - Order By Clause
- SQL - Group By Clause
- SQL - Having Clause
- SQL - AND & OR
- SQL - BOOLEAN (BIT) Operator
- SQL - LIKE Operator
- SQL - IN Operator
- SQL - ANY, ALL Operators
- SQL - EXISTS Operator
- SQL - CASE
- SQL - NOT Operator
- SQL - NOT EQUAL
- SQL - IS NULL
- SQL - IS NOT NULL
- SQL - NOT NULL
- SQL - BETWEEN Operator
- SQL - UNION Operator
- SQL - UNION vs UNION ALL
- SQL - INTERSECT Operator
- SQL - EXCEPT Operator
- SQL - Aliases
- SQL Joins
- SQL - Using Joins
- SQL - Inner Join
- SQL - Left Join
- SQL - Right Join
- SQL - Cross Join
- SQL - Full Join
- SQL - Self Join
- SQL - Delete Join
- SQL - Update Join
- SQL - Left Join vs Right Join
- SQL - Union vs Join
- SQL Keys
- SQL - Unique Key
- SQL - Primary Key
- SQL - Foreign Key
- SQL - Composite Key
- SQL - Alternate Key
- SQL Indexes
- SQL - Indexes
- SQL - Create Index
- SQL - Drop Index
- SQL - Show Indexes
- SQL - Unique Index
- SQL - Clustered Index
- SQL - Non-Clustered Index
- Advanced SQL
- SQL - Wildcards
- SQL - Comments
- SQL - Injection
- SQL - Hosting
- SQL - Min & Max
- SQL - Null Functions
- SQL - Check Constraint
- SQL - Default Constraint
- SQL - Stored Procedures
- SQL - NULL Values
- SQL - Transactions
- SQL - Sub Queries
- SQL - Handling Duplicates
- SQL - Using Sequences
- SQL - Auto Increment
- SQL - Date & Time
- SQL - Cursors
- SQL - Common Table Expression
- SQL - Group By vs Order By
- SQL - IN vs EXISTS
- SQL - Database Tuning
- SQL Function Reference
- SQL - Date Functions
- SQL - String Functions
- SQL - Aggregate Functions
- SQL - Numeric Functions
- SQL - Text & Image Functions
- SQL - Statistical Functions
- SQL - Logical Functions
- SQL - Cursor Functions
- SQL - JSON Functions
- SQL - Conversion Functions
- SQL - Datatype Functions
- SQL Useful Resources
- SQL - Questions and Answers
- SQL - Quick Guide
- SQL - Useful Functions
- SQL - Useful Resources
- SQL - Discussion
- Selected Reading
- UPSC IAS Exams Notes
- Developer's Best Practices
- Questions and Answers
- Effective Resume Writing
- HR Interview Questions
- Computer Glossary
- Who is Who
SQL - IS NULL
The "IS NULL" operator in SQL is used to check if a column has a NULL value. It returns true if the column value is NULL and false if it is not.
A field with a NULL value denotes that it has no value. It is possible to create a new record or update an existing record without providing a value for a field in a table. If we do so, the field will then be saved with the value NULL.
Comparison operators like =, <, or <> cannot be used to check for NULL values. Instead, we use the following operators.
- Is null
- Is not null (negation of NULL values)
Null value in SQL
SQL does not permit leaving any field of a column without value. logically, table columns without values are empty fields. In reality, fields having an unspecified value are regarded as NULL. When we don't enter anything into a table cell, SQL assumes that there is a value that, at this time, is unknown but might one day be known and placed in this field.
Syntax
Following is the syntax for null value −
SELECT column_name1, column_name2, column_name3, ... , column_nameN FROM table_name WHERE column_nameN = NULL
The IS NULL Operator
SQL IS NULL is a logical operator that enables you to filter out rows with missing data from your results. Null values, or cells without any data, can appear in some tables. IS NULL allows you to choose rows in a given column that are empty.
Syntax
Following is the syntax for IS NULL −
SELECT column_name1, column_name2, column_name3, ... , column_nameN FROM table_name WHERE column_nameN IS NULL
Example
Let’s consider a table named "Fruit" that we are going to create in our database and which contains some null values in the fields. Execute the below query to create a table.
SQL> CREATE TABLE Fruit ( ID INT NOT NULL, NAME VARCHAR (20) NOT NULL, ADDRESS CHAR (25), PRICE DECIMAL (18, 2), PRIMARY KEY (ID) );
Now we are going to populate the above-created table by using the below query.
SQL> INSERT INTO Fruit (ID,NAME,ADDRESS,PRICE) VALUES (1, 'Apple', 'Shimla', 2000.00 ); INSERT INTO Fruit (ID,NAME,ADDRESS,PRICE) VALUES (2, 'Mango',NULL, 3000.00 ); INSERT INTO Fruit (ID,NAME,ADDRESS,PRICE) VALUES (3, 'Orange',NULL, 4000.00 ); INSERT INTO Fruit (ID,NAME,ADDRESS,PRICE) VALUES (4, 'Banana', 'AP',NULL); INSERT INTO Fruit (ID,NAME,ADDRESS,PRICE) VALUES (5, 'JackFruit', 'Ooty',NULL);
Verification
To check whether the table is created or not, let’s execute the below query.
SQL> SELECT * FROM Fruit;
On executing it, it will display a table as shown below −
+----+-----------+---------+---------+ | ID | NAME | ADDRESS | PRICE | +----+-----------+---------+---------+ | 1 | Apple | Shimla | 2000.00 | | 2 | Mango | NULL | 3000.00 | | 3 | Orange | NULL | 4000.00 | | 4 | Banana | AP | NULL | | 5 | JackFruit | Ooty | NULL | +----+-----------+---------+---------+
IS NULL with SELECT statement
We can use IS NULL operator with a SELECT statement to filter rows based on whether a particular column contains a NULL value or not.
Example
In the following query, we are going to show how the IS NULL condition is going to be used to select rows if the specified field is NULL.
SQL> SELECT * FROM Fruit WHERE ADDRESS IS NULL;
Output
On executing the above query, it will generate an output as shown below −
+----+--------+---------+---------+ | ID | NAME | ADDRESS | PRICE | +----+--------+---------+---------+ | 2 | Mango | NULL | 3000.00 | | 3 | Orange | NULL | 4000.00 | +----+--------+---------+---------+
IS NULL with COUNT() function
We can also use the IS NULL operator with the COUNT() function in SQL to count the number of rows that contain NULL values in a particular column. This function is utilized along with the SQL SELECT command.
Syntax
Following is the syntax for COUNT() function −
SELECT COUNT(column_name) FROM table_name WHERE condition;
Example
The following query determines the count of rows have a blank field (NULL) in Price column.
SQL> SELECT COUNT(*) FROM Fruit WHERE PRICE IS NULL;
Output
On executing the above query, it will generate an output as shown below −
+----------+ | COUNT(*) | +----------+ | 2 | +----------+
IS NULL with UPDATE statement
We can use the UPDATE statement with the "IS NULL" operator in SQL to set the value of a column to NULL for all rows that meet a certain condition.
Example
Consider the following table "fruit" in our database and run the following query to see how the update statement is used.
SQL> UPDATE Fruit SET PRICE= 1000 WHERE PRICE IS NULL;
Verification
To check whether the table has been updated or not, execute the below query.
SQL> SELECT * FROM Fruit;
On executing the above query, it will generate the following output as shown below −
+----+-----------+---------+---------+ | ID | NAME | ADDRESS | PRICE | +----+-----------+---------+---------+ | 1 | Apple | Shimla | 2000.00 | | 2 | Mango | NULL | 3000.00 | | 3 | Orange | NULL | 4000.00 | | 4 | Banana | AP | 1000.00 | | 5 | JackFruit | Ooty | 1000.00 | +----+-----------+---------+---------+
SQL IS NULL with DELETE statement
We can also use the DELETE statement with IS NULL operator to delete all rows that contain NULL values in a particular column.
Example
Execute the below query to observe how we are going to use the delete statement with is null.
SQL> DELETE FROM Fruit WHERE ADDRESS IS NULL;
Verification
Execute the below query to check whether the table has been changed or not.
SQL> SELECT * FROM Fruit;
On executing the above query, it will generate the following output as shown below −
+----+-----------+---------+---------+ | ID | NAME | ADDRESS | PRICE | +----+-----------+---------+---------+ | 1 | Apple | Shimla | 2000.00 | | 4 | Banana | AP | 1000.00 | | 5 | JackFruit | Ooty | 1000.00 | +----+-----------+---------+---------+